Mastering Dart Classes: A Comprehensive Guide for Developers
Written on
Chapter 1: Understanding Classes in Dart
Classes are a cornerstone of Dart and Flutter development, forming the basis of object-oriented programming. They provide encapsulation, inheritance, and polymorphism, which are critical for creating scalable and maintainable applications. This guide will explore the various aspects of classes in Dart, including fundamental principles, advanced topics, practical examples, and best practices.
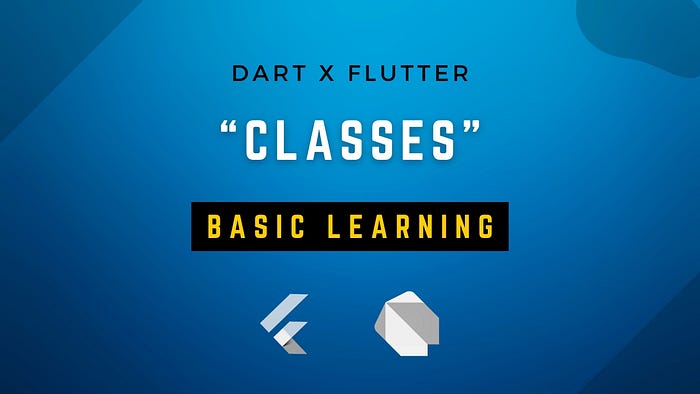
Outline of This Article
- Introduction to Classes in Dart
- Utilizing Class Members
- Working with Constructors
- Determining an Object's Type
- Instance Variables Explained
- Implicit Interfaces in Dart
- Class Variables and Methods
- Conclusion
- FAQs
Introduction
Dart is an object-oriented language that heavily relies on classes. A solid understanding of classes is essential for mastering Dart and Flutter development. In this section, we will examine the key components of classes, how to effectively utilize them, and how to create objects.
Classes in Dart
Dart adopts mixin-based inheritance, allowing class bodies to be reused across various hierarchies. Every class, apart from Null, is a descendant of the Object class. Extension methods enable the addition of new functionalities to a class without changing its existing structure, while class modifiers manage how libraries can subclass a class.
Using Class Members
Objects consist of members, which include functions and data. Methods correspond to functions, while instance variables represent data. To call a method on an object, the dot (.) notation is used:
var p = Point(2, 2);
// Access the y value.
assert(p.y == 2);
// Call distanceTo() on p.
double distance = p.distanceTo(Point(4, 4));
To prevent exceptions when the object may be null, use the ?. operator:
// If p is non-null, assign its y value to a variable.
var a = p?.y;
Working with Constructors
Constructors are special functions used to create objects. They can be named, such as ClassName or ClassName.identifier:
var p1 = Point(2, 2);
var p2 = Point.fromJson({'x': 1, 'y': 2});
You can also use the optional new keyword:
var p1 = new Point(2, 2);
var p2 = new Point.fromJson({'x': 1, 'y': 2});
Constant constructors enable the creation of compile-time constants with the const keyword:
var p = const ImmutablePoint(2, 2);
Getting an Object's Type
To determine an object's type at runtime, use the runtimeType property:
print('The type of a is ${a.runtimeType}');
For production environments, it’s advisable to utilize type test operators for more stable type checking.
Instance Variables Explained
Instance variables reflect an object's state and can be declared with or without initial values:
class Point {
double? x; // Starts as null.
double? y; // Starts as null.
double z = 0; // Starts as 0.
}
Non-late instance variables initialized upon declaration will have their values set at instance creation. Non-nullable variables must be initialized:
class Point {
double? x; // Starts as null.
double? y; // Starts as null.
}
void main() {
var point = Point();
point.x = 4; // Use the setter for x.
assert(point.x == 4); // Use the getter for x.
assert(point.y == null); // Default values are null.
}
Implicit Interfaces in Dart
Dart's implicit interfaces mean every class automatically defines an interface that includes all its instance members. When a class implements one or more interfaces, it declares them using the implements clause, allowing the creation of classes that mimic another class's API without inheriting its implementation:
class Person {
final String _name;
Person(this._name);
String greet(String who) => 'Hello, $who. I am $_name.';
}
class Impostor implements Person {
String get _name => '';
String greet(String who) => 'Hi $who. Do you know who I am?';
}
String greetBob(Person person) => person.greet('Bob');
void main() {
print(greetBob(Person('Kathy')));
print(greetBob(Impostor()));
}
This example illustrates how the Impostor class implements the Person interface without directly inheriting its implementation.
Class Variables and Methods
Dart employs the static keyword for class-wide variables and methods. Static variables serve as class-wide states and constants, while static methods operate independently of instance-specific data. They can be called directly on the class:
- Static Variables: Initialized on first use:
class Queue {
static const initialCapacity = 16;
// ...
}
void main() {
assert(Queue.initialCapacity == 16);
}
- Static Methods: Operate at the class level and lack access to instance-specific data:
import 'dart:math';
class Point {
double x, y;
Point(this.x, this.y);
static double distanceBetween(Point a, Point b) {
var dx = a.x - b.x;
var dy = a.y - b.y;
return sqrt(dx * dx + dy * dy);
}
}
void main() {
var a = Point(2, 2);
var b = Point(4, 4);
var distance = Point.distanceBetween(a, b);
assert(2.8 < distance && distance < 2.9);
print(distance);
}
It’s often better to use top-level functions for common utilities instead of static methods.
Conclusion
In this guide, we examined the essentials of classes in Dart, including their creation, usage, constructors, type determination, and instance variables. We also explored implicit interfaces, demonstrating how Dart allows classes to implement APIs of others without direct inheritance, along with static variables and methods which facilitate the definition of class-wide constants and functionalities.
FAQs
Why are classes crucial in Dart and Flutter development?
Classes are fundamental for object-oriented programming, providing encapsulation, inheritance, and polymorphism, which enhance code organization and maintainability.
How can I access methods and instance variables in Dart classes?
You can access methods and instance variables using dot (.) notation, and handle null scenarios with the ?. operator to avoid exceptions.
What are named constructors, and how do they differ from default constructors in Dart?
Named constructors allow for alternative object creation methods that enhance clarity and flexibility, while default constructors are automatically provided.
How can I determine an object's type in Dart?
Use the runtimeType property, but prefer type test operators for robust type checking in production.
What role do instance variables play in Dart?
Instance variables signify an object's state, with distinctions between nullable and non-nullable variables essential for proper initialization.
The Complete Dart & Flutter Developer Course | Full Tutorial For Beginners to Advanced
This extensive course covers everything from the basics to advanced topics in Dart and Flutter, ideal for both beginners and experienced developers.
The Best & Most Complete Dart Course - Visualize, Learn and Practice all Dart Language Concepts!
This course provides an in-depth exploration of Dart, focusing on practical examples and a thorough understanding of all language concepts.