Mastering DRY Principles in React: 5 Essential Techniques
Written on
Chapter 1: Introduction to the DRY Principle
Programming is often viewed as a craft rather than merely a job, and this perspective is well-founded. As developers, we should strive for the highest quality in the software we create. One of the key principles guiding this pursuit is the DRY principle: "Don't Repeat Yourself." In this article, I will introduce five methods I personally utilize to minimize code duplication in my React projects.
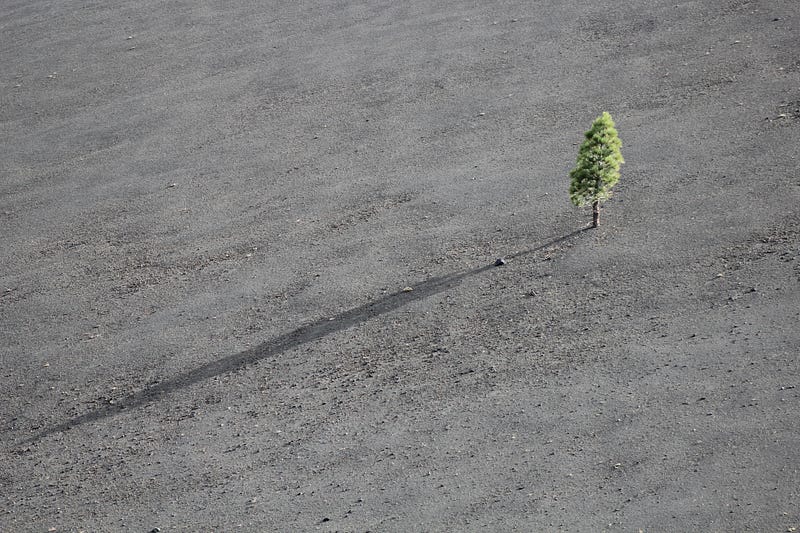
Chapter 2: Techniques to Avoid Code Duplication
Section 2.1: Streamlining HTTP Calls
Fetching data from remote sources is a fundamental task in modern React applications. However, beginners often make common mistakes during this process. Here's a typical progression:
Step 1: Initial Data Fetching
Many new developers fetch data directly within their components, a method often presented in beginner tutorials.
Step 2: Logic Abstraction
Next, developers typically move this logic into a centralized store management system, like Redux or Context. Yet, this often leads to repeated code.
How to Eliminate Redundancies:
- First Approach: Create a dedicated HttpUtility class or a custom useHttp hook to handle all HTTP requests. Libraries like Axios can simplify remote calls, but managing loading states and errors can become cumbersome.
- Enhanced Strategy: Invest time learning about libraries such as React Query, which offers powerful features suitable for applications of various sizes.
Description: This video explains effective methods for writing clean code in React, emphasizing the importance of adhering to SOLID principles.
Section 2.2: Effective Error Handling
From my observations, error handling is often mishandled. Triggering toast notifications in each component is generally not advisable. Common scenarios requiring user feedback include:
Errors During HTTP Calls
Utilizing middleware to intercept actions before they reach the Redux store is effective for managing errors. Capture errors from HTTP responses and display notifications.
Component Logic Errors
Implementing an ErrorBoundary can help catch component loading errors, reducing the need for null checks throughout your code.
Validation Errors
When form validation fails, it’s essential to provide feedback. Consider using libraries like Yup for automatic validation error handling, or utilize react-hook-form for streamlined management.
Section 2.3: Component Composition
One of React's most powerful features is its ability to create reusable components. Beginners often overlook this aspect, but breaking down components into smaller, manageable pieces has several benefits:
- Enhanced code quality and readability
- Reduction of duplicate UI components across the project
Section 2.4: Leveraging Custom Hooks
I am a strong advocate for using hooks, and I believe new developers should embrace them early on. When you find yourself repeating logic, React offers solutions like Higher-Order Components (HOCs). However, hooks provide a cleaner way to achieve this abstraction.
Section 2.5: Avoiding Duplicate Styles
Styling can be a significant challenge, especially for beginners. My evolution as a developer in this area progressed as follows:
Step 1: Using CSS Files
While CSS files are standard, poorly organized styles can lead to duplicated logic.
Step 2: Transitioning to Sass
Sass allows for more modular CSS writing, enabling code reuse through mixins and variables, but it can still be complex to manage.
Finally, I discovered styled-components, which simplifies style reuse and enhances component readability, eliminating clutter from my code.
Description: This video offers advanced examples of applying SOLID principles in React, focusing on writing clean, maintainable code.
Conclusion
These five techniques can significantly help you adhere to the DRY principle within your React projects. While these methods aren't foolproof, grasping how they work can greatly enhance your coding journey. For those interested in more advanced best practices, consider checking out additional resources.
Feel free to connect with me on LinkedIn or visit my personal website for more insights!