Mastering Smooth Curves with JavaScript and HTML5 Canvas
Written on
Chapter 1: Introduction to Smooth Curves
Sometimes, you may want to illustrate a smooth curve on a webpage that passes through several designated points. In this guide, we will explore how to effectively draw a smooth curve using JavaScript's HTML5 canvas.
Section 1.1: The quadraticCurveTo Method
A useful approach for creating curves in the HTML5 canvas is through the quadraticCurveTo method associated with the canvas context object. To begin, you will first need to set up the HTML structure. Here’s an example:
<canvas width="200" height="200"></canvas>
Next, you can implement the following JavaScript code:
const points = [
{ x: 1, y: 1 },
{ x: 20, y: 30 },
{ x: 30, y: 40 },
{ x: 40, y: 20 },
{ x: 50, y: 60 }
];
const canvas = document.querySelector('canvas');
const ctx = canvas.getContext("2d");
ctx.beginPath();
ctx.moveTo(points[0].x, points[0].y);
for (const point of points) {
const xMid = (point.x + point.x) / 2;
const yMid = (point.y + point.y) / 2;
const cpX1 = (xMid + point.x) / 2;
const cpX2 = (xMid + point.x) / 2;
ctx.quadraticCurveTo(cpX1, point.y, xMid, yMid);
ctx.quadraticCurveTo(cpX2, point.y, point.x, point.y);
}
ctx.stroke();
This code snippet defines an array of points with their respective x and y coordinates. After selecting the canvas element with document.querySelector, we retrieve its context using getContext. We initiate the drawing process with beginPath, move to the starting point using moveTo, and subsequently draw the curve using quadraticCurveTo.
Finally, we use stroke to render the line on the canvas.
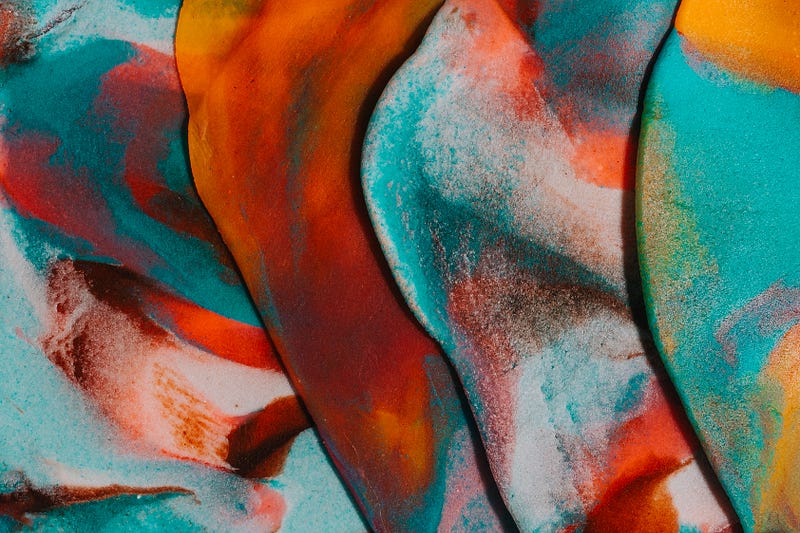
Section 1.2: Conclusion
In summary, the quadraticCurveTo method provided by the canvas context object is instrumental in drawing smooth curves that connect multiple points.
Chapter 2: Video Tutorials
For a more visual approach, check out these helpful video tutorials:
The first video, How to Draw a Smooth Curve Through N Points Using JavaScript HTML5 Canvas, offers a step-by-step guide on implementing this technique.
Additionally, the second video, Canvas - Lines and Curves - Episode 3, delves deeper into drawing techniques with the HTML5 canvas.
For more resources and to enhance your skills, consider subscribing to our free weekly newsletter at PlainEnglish.io. Follow us on social media platforms like Twitter, LinkedIn, YouTube, and Discord. If you're interested in growth hacking strategies, check out Circuit.